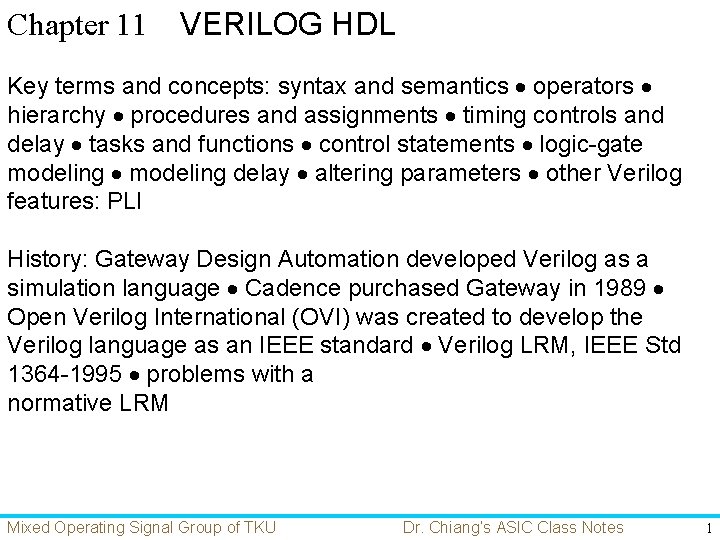
Hierarchical Identifiers
Hierarchical path names are based on the top module identifier followed by module instant identifiers, separated by periods.
This is useful basically when we want to see the signal inside a lower module, or want to force a value inside an internal module. The example below shows how to monitor the value of an internal module signal.
Example
1 //-----------------------------------------------------
2 // This is simple adder Program
3 // Design Name : adder_hier
4 // File Name : adder_hier.v
5 // Function : This program shows verilog hier path works
6 // Coder : Deepak
7 //-----------------------------------------------------
8 `include "addbit.v"
9 module adder_hier (
10 result , // Output of the adder
11 carry , // Carry output of adder
12 r1 , // first input
13 r2 , // second input
14 ci // carry input
15 );
16
17 // Input Port Declarations
18 input [3:0] r1 ;
19 input [3:0] r2 ;
20 input ci ;
21
22 // Output Port Declarations
23 output [3:0] result ;
24 output carry ;
25
26 // Port Wires
27 wire [3:0] r1 ;
28 wire [3:0] r2 ;
29 wire ci ;
30 wire [3:0] result ;
31 wire carry ;
32
33 // Internal variables
34 wire c1 ;
35 wire c2 ;
36 wire c3 ;
37
38 // Code Starts Here
39 addbit u0 (r1[0],r2[0],ci,result[0],c1);
40 addbit u1 (r1[1],r2[1],c1,result[1],c2);
41 addbit u2 (r1[2],r2[2],c2,result[2],c3);
42 addbit u3 (r1[3],r2[3],c3,result[3],carry);
43
44 endmodule // End Of Module adder
45
46 module tb();
47
48 reg [3:0] r1,r2;
49 reg ci;
50 wire [3:0] result;
51 wire carry;
52
53 // Drive the inputs
54 initial begin
55 r1 = 0;
56 r2 = 0;
57 ci = 0;
58 #10 r1 = 10;
59 #10 r2 = 2;
60 #10 ci = 1;
61 #10 $display("+--------------------------------------------------------+");
62 $finish;
63 end
64
65 // Connect the lower module
66 adder_hier U (result,carry,r1,r2,ci);
67
68 // Hier demo here
69 initial begin
70 $display("+--------------------------------------------------------+");
71 $display("| r1 | r2 | ci | u0.sum | u1.sum | u2.sum | u3.sum |");
72 $display("+--------------------------------------------------------+");
73 $monitor("| %h | %h | %h | %h | %h | %h | %h |",
74 r1,r2,ci, tb.U.u0.sum, tb.U.u1.sum, tb.U.u2.sum, tb.U.u3.sum);
75 end
76
77 endmodule
+--------------------------------------------------------+
| r1 | r2 | ci | u0.sum | u1.sum | u2.sum | u3.sum |
+--------------------------------------------------------+
| 0 | 0 | 0 | 0 | 0 | 0 | 0 |
| a | 0 | 0 | 0 | 1 | 0 | 1 |
| a | 2 | 0 | 0 | 0 | 1 | 1 |
| a | 2 | 1 | 1 | 0 | 1 | 1 |
+--------------------------------------------------------+
Data Types
Verilog Language has two primary data types:
- Nets - represent structural connections between components.
- Registers - represent variables used to store data.
Every signal has a data type associated with it:
- Explicitly declared with a declaration in your Verilog code.
- Implicitly declared with no declaration when used to connect structural building blocks in your code. Implicit declaration is always a net type "wire" and is one bit wide.
Types of Nets
Each net type has a functionality that is used to model different types of hardware (such as PMOS, NMOS, CMOS, etc)
Net Data Type | Functionality |
wire, tri | Interconnecting wire - no special resolution function |
wor, trior | Wired outputs OR together (models ECL) |
wand, triand | Wired outputs AND together (models open-collector) |
tri0, tri1 | Net pulls-down or pulls-up when not driven |
supply0, supply1 | Net has a constant logic 0 or logic 1 (supply strength) |
trireg | Retains last value, when driven by z (tristate). |