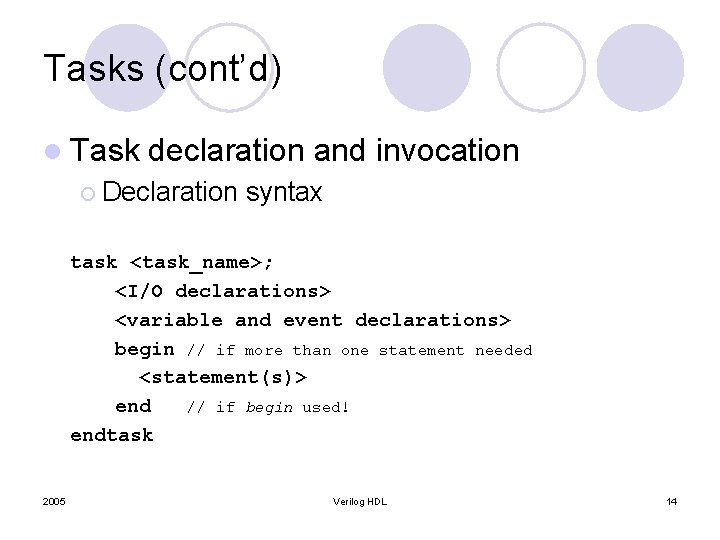
Example - wor
1 module test_wor();
2
3 wor a;
4 reg b, c;
5
6 assign a = b;
7 assign a = c;
8
9 initial begin
10 $monitor("%g a = %b b = %b c = %b", $time, a, b, c);
11 #1 b = 0;
12 #1 c = 0;
13 #1 b = 1;
14 #1 b = 0;
15 #1 c = 1;
16 #1 b = 1;
17 #1 b = 0;
18 #1 $finish;
19 end
20
21 endmodule
Simulator Output
0 a = x b = x c = x
1 a = x b = 0 c = x
2 a = 0 b = 0 c = 0
3 a = 1 b = 1 c = 0
4 a = 0 b = 0 c = 0
5 a = 1 b = 0 c = 1
6 a = 1 b = 1 c = 1
7 a = 1 b = 0 c = 1
Example – wand
1 module test_wand();
2
3 wand a;
4 reg b, c;
5
6 assign a = b;
7 assign a = c;
8
9 initial begin
10 $monitor("%g a = %b b = %b c = %b", $time, a, b, c);
11 #1 b = 0;
12 #1 c = 0;
13 #1 b = 1;
14 #1 b = 0;
15 #1 c = 1;
16 #1 b = 1;
17 #1 b = 0;
18 #1 $finish;
19 end
20
21 endmodule
Simulator Output |
0 a = x b = x c = x
1 a = 0 b = 0 c = x
2 a = 0 b = 0 c = 0
3 a = 0 b = 1 c = 0
4 a = 0 b = 0 c = 0
5 a = 0 b = 0 c = 1
6 a = 1 b = 1 c = 1
7 a = 0 b = 0 c = 1
Example - tri
1 module test_tri();
2
3 tri a;
4 reg b, c;
5
6 assign a = (b) ? c : 1'bz;
7
8 initial begin
9 $monitor("%g a = %b b = %b c = %b", $time, a, b, c);
10 b = 0;
11 c = 0;
12 #1 b = 1;
13 #1 b = 0;
14 #1 c = 1;
15 #1 b = 1;
16 #1 b = 0;
17 #1 $finish;
18 end
19
20 endmodule
Simulator Output |
0 a = z b = 0 c = 0 1 a = 0 b = 1 c = 0 2 a = z b = 0 c = 0 3 a = z b = 0 c = 1 4 a = 1 b = 1 c = 1 5 a = z b = 0 c = 1 |
Example – trireg
1 module test_trireg();
2
3 trireg a;
4 reg b, c;
5
6 assign a = (b) ? c : 1'bz;
7
8 initial begin
9 $monitor("%g a = %b b = %b c = %b", $time, a, b, c);
10 b = 0;
11 c = 0;
12 #1 b = 1;
13 #1 b = 0;
14 #1 c = 1;
15 #1 b = 1;
16 #1 b = 0;
17 #1 $finish;
18 end
19
20 endmodule
Simulator Output
0 a = x b = 0 c = 0
1 a = 0 b = 1 c = 0
2 a = 0 b = 0 c = 0
3 a = 0 b = 0 c = 1
4 a = 1 b = 1 c = 1
5 a = 1 b = 0 c = 1
Register Data Types
- Registers store the last value assigned to them until another assignment statement changes their value.
- Registers represent data storage constructs.
- You can create regs arrays called memories.
- register data types are used as variables in procedural blocks.
- A register data type is required if a signal is assigned a value within a procedural block
- Procedural blocks begin with keyword initial and always.
| ||||||||||
Note : Of all register types, reg is the one which is most widely used |
Strings
A string is a sequence of characters enclosed by double quotes and all contained on a single line. Strings used as operands in expressions and assignments are treated as a sequence of eight-bit ASCII values, with one eight-bit ASCII value representing one character. To declare a variable to store a string, declare a register large enough to hold the maximum number of characters the variable will hold. Note that no extra bits are required to hold a termination character; Verilog does not store a string termination character. Strings can be manipulated using the standard operators.
When a variable is larger than required to hold a value being assigned, Verilog pads the contents on the left with zeros after the assignment. This is consistent with the padding that occurs during assignment of non-string values. | ||
Certain characters can be used in strings only when preceded by an introductory character called an escape character. The following table lists these characters in the right-hand column together with the escape sequence that represents the character in the left-hand column. | ||
Special Characters in Strings | ||
Character | Description | . |
\n | New line character | . |
\t | Tab character | . |
\\ | Backslash (\) character | . |
\" | Double quote (") character | . |
\ddd | A character specified in 1-3 octal digits (0 <= d <= 7) | . |
%% | Percent (%) character | . |
Example
1 //-----------------------------------------------------
2 // Design Name : strings
3 // File Name : strings.v
4 // Function : This program shows how string
5 // can be stored in reg
6 // Coder : Deepak Kumar Tala
7 //-----------------------------------------------------
8 module strings();
9 // Declare a register variable that is 21 bytes
10 reg [8*21:0] string ;
11
12 initial begin
13 string = "This is sample string";
14 $display ("%s \n", string);
15 end
16
17 endmodule
Bạn Có Đam Mê Với Vi Mạch hay Nhúng - Bạn Muốn Trau Dồi Thêm Kĩ Năng
Mong Muốn Có Thêm Cơ Hội Trong Công Việc
Và Trở Thành Một Người Có Giá Trị Hơn